Mollie Components
Overview
Mollie Components is a set of Javascript APIs that allow you to add the fields needed for credit card holder data to your own checkout, in a way that is fully PCI-DSS SAQ-A compliant.
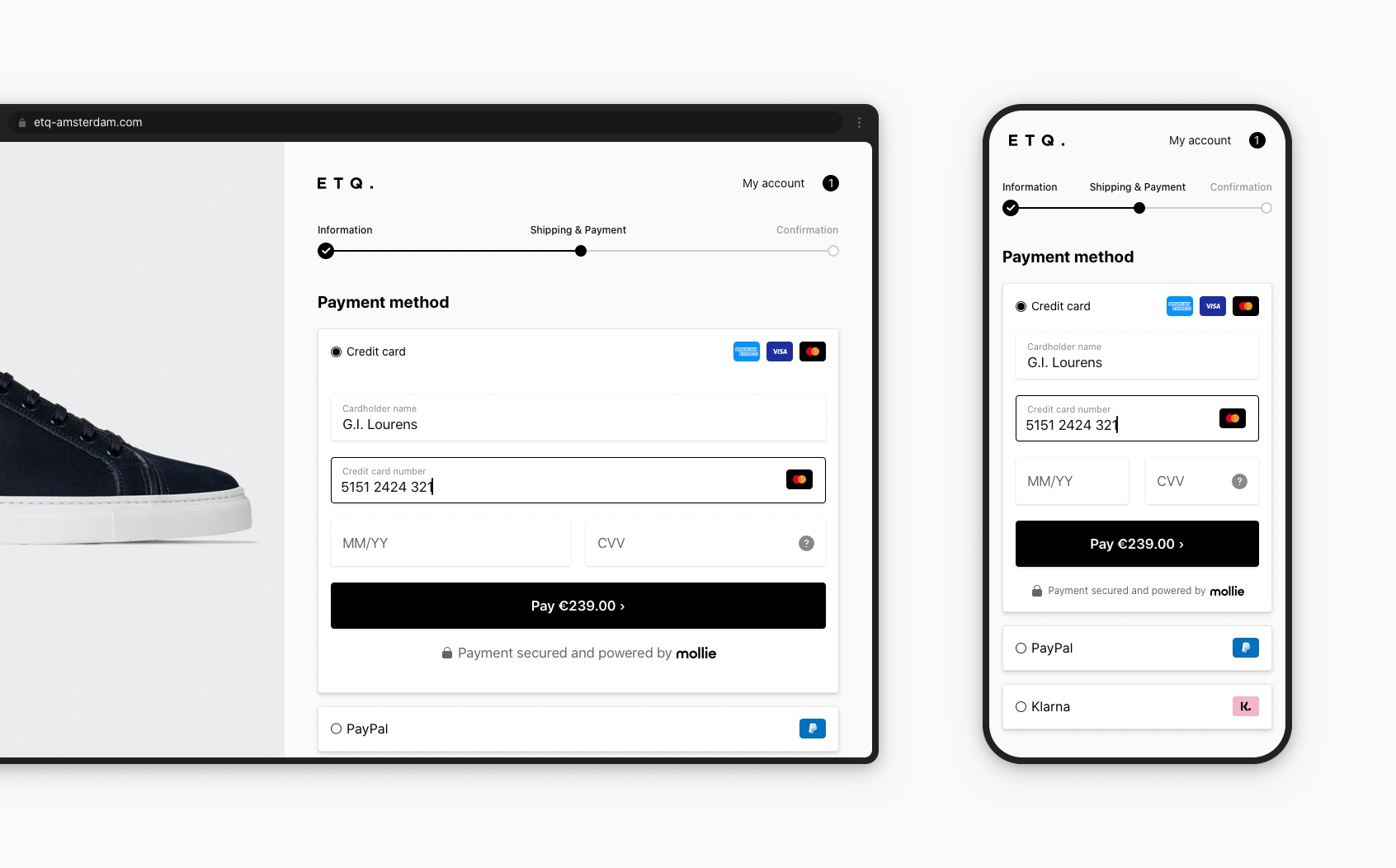
At a high level, it works by using a Javascript API, dubbed Mollie.js, to add fields to your checkout that your customer will use to enter their credit card details, such as their card number.
Mollie Components does not give you access to the card holder data. Instead, when the checkout is submitted, you use Mollie Components to exchange the card holder data for a cardToken
which you can use with the Create payment or Create order endpoints.
Once you have created a payment or order, you should redirect your customer to the URL in the _links.checkout
property from the response. This link is where your customer can perform the 3-D Secure authentication. If the customer authenticates successfully, the payment is completed.
Implementation steps
Follow these steps to implement Mollie Components in your checkout:
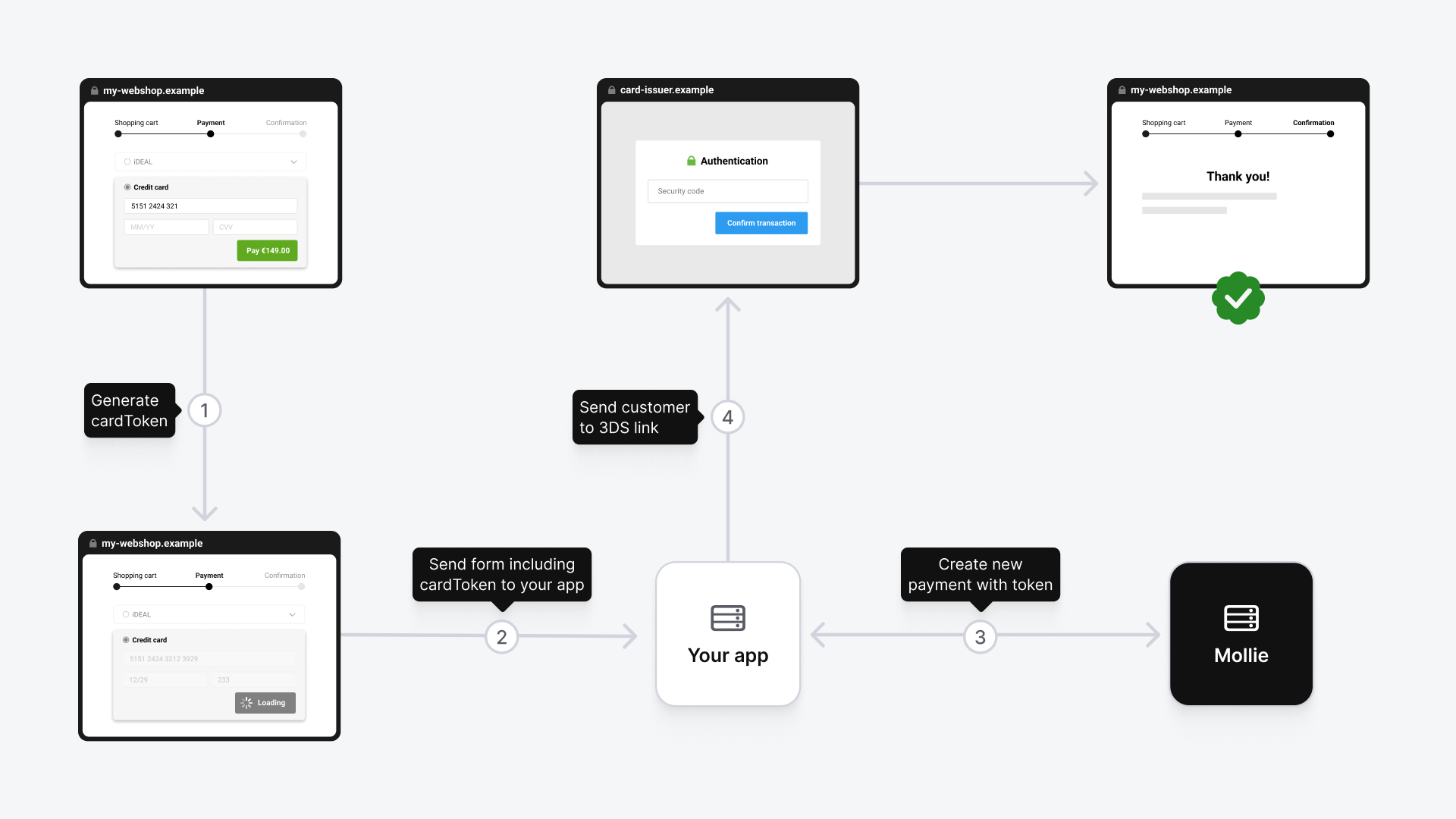
- Add Mollie.js to your checkout.
- Initialize the
Mollie
object. - Create and mount the four Components for the four credit card fields (card holder, card number, expiry date and CVC). This will add the fields to your checkout.
- Add a
submit
event listener to your form to retrieve thecardToken
when your customer has completed the checkout form. - Send the
cardToken
to your back end, by adding it to your form. - From your back end, create a credit card payment or order with the
cardToken
using the Create payment endpoint or Create order endpoint respectively. - Redirect the shopper to the URL returned by our API for 3-D Secure authentication.
Mollie has created example implementations you can use to get started.
Add Mollie.js to your checkout
Start by including Mollie.js into your project. It should be added just before the </body>
tag.
The JavaScript file is located at https://js.mollie.com/v1/mollie.js
.
<html>
<head>
<title>My Checkout</title>
</head>
<body>
<script src="https://js.mollie.com/v1/mollie.js"></script>
</body>
</html>
Note
If you are using Content Security Policy, you should whitelist the
js.mollie.com
domain (e.g.script-src js.mollie.com
) and allow inline styles (e.g.style-src 'unsafe-inline'
). We recommend using a strict CSP on your checkout.
Initialize the Mollie object
First, you need the ID of the profile that you want to use This can be found on the Developers - API-keys page in the Web app or retrieved programmatically using the Get current profile endpoint.
After the script has loaded you can use the Mollie(profileId[, options]) function. This will return an object that you can use for creating the four Components your customer will use to enter their card holder data.
var mollie = Mollie('pfl_3RkSN1zuPE', { locale: 'nl_NL', testmode: false });
Note
Be aware the profile ID is not your API key. Your API key is private and should never be used in a browser context. The profile ID starts with
pfl_,
where as API keys start withlive_
ortest_.
Create and mount Mollie Components
After initialising the Mollie object, you can start to consume the shopperβs data. There are two ways to do this:
- Use the card component to implement our out-of-the-box solution.
- Use Mollie Components to build your own solution that covers your specific use cases.
Option 1: Card component
The card component is a collection of all mandatory fields needed to create an embedded card form. With this component, you can abstract your implementation from the DOM. This makes it easier to implement while covering most use cases. You can create the card component using the mollie.createComponent(type[, options]) and mount it in your checkout using the component.mount(targetElement).
<form>
<div id="card"></div>
</form>
var cardComponent = mollie.createComponent('card');
cardComponent.mount('#card');
Translated error messages will be rendered within the DOM automatically.
To customize the card component, see mollie.createComponent(type[, options]).
To add styling to the card component, see Styling.
Option 2: Mollie Components
Mollie Components are individual mandatory components out of which you can create a card form. You can create them using the mollie.createComponent(type[, options]) and mount them in your checkout using the component.mount(targetElement). This will add the input fields to your checkout and make them visible to your customer.
<form>
<div id="card-number"></div>
<div id="card-number-error"></div>
<div id="card-holder"></div>
<div id="card-holder-error"></div>
<div id="expiry-date"></div>
<div id="expiry-date-error"></div>
<div id="verification-code"></div>
<div id="verification-code-error"></div>
<button type="button">Pay</button>
</form>
var cardNumber = mollie.createComponent('cardNumber');
cardNumber.mount('#card-number');
var cardHolder = mollie.createComponent('cardHolder');
cardHolder.mount('#card-holder');
var expiryDate = mollie.createComponent('expiryDate');
expiryDate.mount('#expiry-date');
var verificationCode = mollie.createComponent('verificationCode');
verificationCode.mount('#verification-code');
To add styling to the Mollie Components, see Styling.
To handle errors in Mollie Components you have to add a change event listener to each component to listen for errors. Displaying the error is up to you. The example below assumes an empty element in which the error can be rendered.
Errors will be localized according to the locale defined when initializing Mollie Components.
var cardNumberError = document.querySelector('#card-number-error');
cardNumber.addEventListener('change', event => {
if (event.error && event.touched) {
cardNumberError.textContent = event.error;
} else {
cardNumberError.textContent = '';
}
});
Add a submit event listener to your form
Add a submit event listener to your form and use the mollie.createToken() function to get the token. You can then place the cardToken
in a hidden input to submit it to your back end, for example:
form.addEventListener('submit', async e => {
e.preventDefault();
var { token, error } = await mollie.createToken();
if (error) {
// Something wrong happened while creating the token. Handle this situation gracefully.
return;
}
// Add token to the form
var tokenInput = document.createElement('input');
tokenInput.setAttribute('type', 'hidden');
tokenInput.setAttribute('name', 'cardToken');
tokenInput.setAttribute('value', token);
form.appendChild(tokenInput);
// Submit form to the server
form.submit();
});
Create a payment or order with the card token
On your back end, you will receive the cardToken
. You need to pass this when creating a payment. Additionally, you should set the method
to creditcard
.
Alternatively, when using the Orders API, you can pass the card token via the payment.cardToken
parameter.
The cardToken
is valid for 1 hour.
Example
curl -X POST https://api.mollie.com/v2/payments \
-H "Authorization: Bearer live_dHar4XY7LxsDOtmnkVtjNVWXLSlXsM" \
-d "method=creditcard" \
-d "amount[currency]=EUR" \
-d "amount[value]=10.00" \
-d "description=Order #12345" \
-d "redirectUrl=https://webshop.example.org/order/12345/" \
-d "webhookUrl=https://webshop.example.org/payments/webhook/" \
-d "cardToken=tkn_UqAvArS3gw"
<?php
$mollie = new \Mollie\Api\MollieApiClient();
$mollie->setApiKey("live_dHar4XY7LxsDOtmnkVtjNVWXLSlXsM");
$payment = $mollie->payments->create([
"method" => "creditcard",
"amount" => [
"currency" => "EUR",
"value" => "10.00"
],
"description" => "Order #12345",
"redirectUrl" => "https://webshop.example.org/order/12345/",
"webhookUrl" => "https://webshop.example.org/payments/webhook/",
"cardToken" => "tkn_UqAvArS3gw",
]);
from mollie.api.client import Client
mollie_client = Client()
mollie_client.set_api_key('live_dHar4XY7LxsDOtmnkVtjNVWXLSlXsM')
payment = mollie_client.payments.create({
'method': 'creditcard',
'amount': {
'currency': 'EUR',
'value': '10.00'
},
'description': 'Order #12345',
'redirectUrl': 'https://webshop.example.org/order/12345/',
'webhookUrl': 'https://webshop.example.org/payments/webhook/',
'cardToken': 'tkn_UqAvArS3gw'
})
require 'mollie-api-ruby'
Mollie::Client.configure do |config|
config.api_key = 'live_dHar4XY7LxsDOtmnkVtjNVWXLSlXsM'
end
payment = Mollie::Payment.create(
method: 'creditcard',
amount: {
currency: 'EUR',
value: '10.00'
},
description: 'Order #12345',
redirect_url: 'https://webshop.example.org/order/12345/',
webhook_url: 'https://webshop.example.org/payments/webhook/',
card_token: 'tkn_UqAvArS3gw'
)
const { createMollieClient } = require('@mollie/api-client');
const mollieClient = createMollieClient({ apiKey: 'live_dHar4XY7LxsDOtmnkVtjNVWXLSlXsM' });
const payment = await mollieClient.payments.create({
method: 'creditcard',
amount: {
currency: 'EUR',
value: '10.00'
},
description: 'Order #12345',
redirectUrl: 'https://webshop.example.org/order/12345/',
webhookUrl: 'https://webshop.example.org/payments/webhook/',
cardToken: 'tkn_UqAvArS3gw'
});
Response
HTTP/1.1 201 Created
Content-Type: application/hal+json
{
"resource": "payment",
"id": "tr_7UhSN1zuXS",
"mode": "test",
"createdAt": "2018-03-20T09:13:37+00:00",
"amount": {
"value": "10.00",
"currency": "EUR"
},
"description": "Order #12345",
"method": null,
"metadata": {
"order_id": "12345"
},
"status": "open",
"isCancelable": false,
"expiresAt": "2018-03-20T09:28:37+00:00",
"profileId": "pfl_3RkSN1zuPE",
"sequenceType": "oneoff",
"details": {
"cardToken": "tkn_UqAvArS3gw"
},
"redirectUrl": "https://webshop.example.org/order/12345/",
"webhookUrl": "https://webshop.example.org/payments/webhook/",
"_links": {
"self": {
"href": "https://api.mollie.com/v2/payments/tr_7UhSN1zuXS",
"type": "application/json"
},
"checkout": {
"href": "https://pay.mollie.com/authenticate/b47ef2ce1d3bea2ddadf3895080d1d4c",
"type": "text/html"
},
"documentation": {
"href": "https://docs.mollie.com/reference/v2/payments-api/create-payment",
"type": "text/html"
}
}
}
Make sure you use the API key that belongs to the same profile you used when initializing the Mollie
object.
It is possible an error occurs when creating the payment. See Handling errors for what to do in such cases.
Redirect the shopper to the 3-D Secure authentication page
You should redirect your customer to the _links.checkout URL
returned by the Create payment endpoint or the Create order endpoint. Your customer can then authenticate themselves with the card issuer.
It is possible an error occurs during or after 3-D Secure authentication. See Handling errors for more information on how to handle these cases.
Browser support
Mollie Components supports the current and previous major release of the following browsers:
- Chrome
- Chrome for Android
- Safari
- Safari iOS
- Opera
- Firefox
- Edge
- The latest release of Microsoft Internet Explorer 11 is supported as well.
If you need to support older browsers, you cannot use Mollie Components.
Updated 10 days ago